Greetings! I'm Aneesh Sreedharan, CEO of 2Hats Logic Solutions. At 2Hats Logic Solutions, we are dedicated to providing technical expertise and resolving your concerns in the world of technology. Our blog page serves as a resource where we share insights and experiences, offering valuable perspectives on your queries.
Slack is the most popular messaging platform for official purposes. In this blog I will explain how we can send messages to the slack public channel or private account through their api’s. First, we need an api user. You could either use an existing slack account or create a new one. While sending messages through the api, the sender name will be this user’s username. So in most cases, creating a new user would be better.
You can login or sign up here (https://slack.com/)
After logging into your account we need to create a Slack App. You can create new App from here (https://api.slack.com/apps)
After creating the new App, it will redirect to the app details page. Here, you can see the app details like App ID, Client ID, Client Secret etc.
Next, go to the OAuth & Permissions menu from the left menu bar to see the Oauth Access Token. We need to add some scopes to the App, we will take a detailed look at scopes next.
Scopes
Basically, scopes are the permissions of the App. Scopes define the API methods an app is allowed to call, which information and capabilities are available on the workspace it’s installed on. To send a message, we need to add some scopes to the App.
• Chat:write:bot – by adding this scope we can send message to the slack as App
• chat:write:user – by adding this scope we can send message to the slack as user
• Users:read – by adding this scope we can view the users in the workspace.
• Users:read.email : – by using this scope we can find a user by email and we will get the details of that user. To send a message to the user we need to get the user id. For this, first we will search the user by email and take the user id from the Api response.
You can add these scopes from the OAuth & Permissions page and you will get all scopes and permission details from here (https://api.slack.com/scopes)
All configurations are done! then let’s see how to send a message.
Send messages to a Channel
For sending requests I am using Guzzle as a client. Guzzle is a PHP HTTP client that makes it easy to send HTTP requests. If you already not installed Guzzle, it can be easily installed by using composer by this command
[code lang=”php”]composer require guzzlehttp/guzzle
[/code]You can use any other http client of your choice
[code lang=”php”]$client = new GuzzleHttp\Client([‘headers’ => [
‘Authorization’ => ‘Bearer OAuth Access Token,
‘Content-Type’ => ‘application/json’,
]]);
$formData = [
‘form_params’ => [
‘channel’ => ‘#channelName’,
‘text’ => ‘Message to be sent’,
‘as_user’ => true,
]];
$response = $client->post(‘https://slack.com/api/chat.postMessage’, $formData)->getBody()->getContents();
$response = json_decode($response, true);
In the above code snippet, the channel parameter defines the channel name to which the message is sent. You can get a full list of parameters that used to send message API from here (https://api.slack.com/methods/chat.postMessage).
Send message response will be like this
Send messages to a user’s private account
[code lang=”php”]$client = new GuzzleHttp\Client([‘headers’ => [
‘Authorization’ => ‘Bearer OAuth Access Token,
‘Content-Type’ => ‘application/json’,
]]);
//Search user by email
$userDetails = $client->get(config(‘https://slack.com/api/[email protected]’)->getBody()->getContents();
$userDetails = json_decode($user, true);
In the above code snippet, enter the user’s email in the email parameter. The search user API response will be something like this
[code lang=”php”]
//If there is user, then send message
if ($userDetails[‘ok’] && !empty($userDetails[‘user’])) {
$formData = [
‘form_params’ => [
‘channel’ => $userDetails[‘user’][‘id’],
‘text’ => ‘Message to be sent’,
‘as_user’ => true,
]];
$response = $client->post(‘https://slack.com/api/chat.postMessage’, $formData)->getBody()->getContents();
$response = json_decode($response, true);
}
Conclusion
In this blog, we have explored how to send messages to Slack public channels and private accounts using the Slack API. We covered the steps involved in creating an API user, setting up an app, and configuring scopes. We also provided code examples for sending messages to channels and users using Guzzle, a popular HTTP client.
By leveraging the Slack API, you can automate tasks, integrate Slack with other applications, and enhance communication within your team. We encourage you to experiment with the API and explore its full potential. Remember to handle errors and edge cases gracefully, and always prioritize clear and concise communication in your messages.
We hope this blog has been helpful! If you have any questions or suggestions, please feel free to leave a comment below.
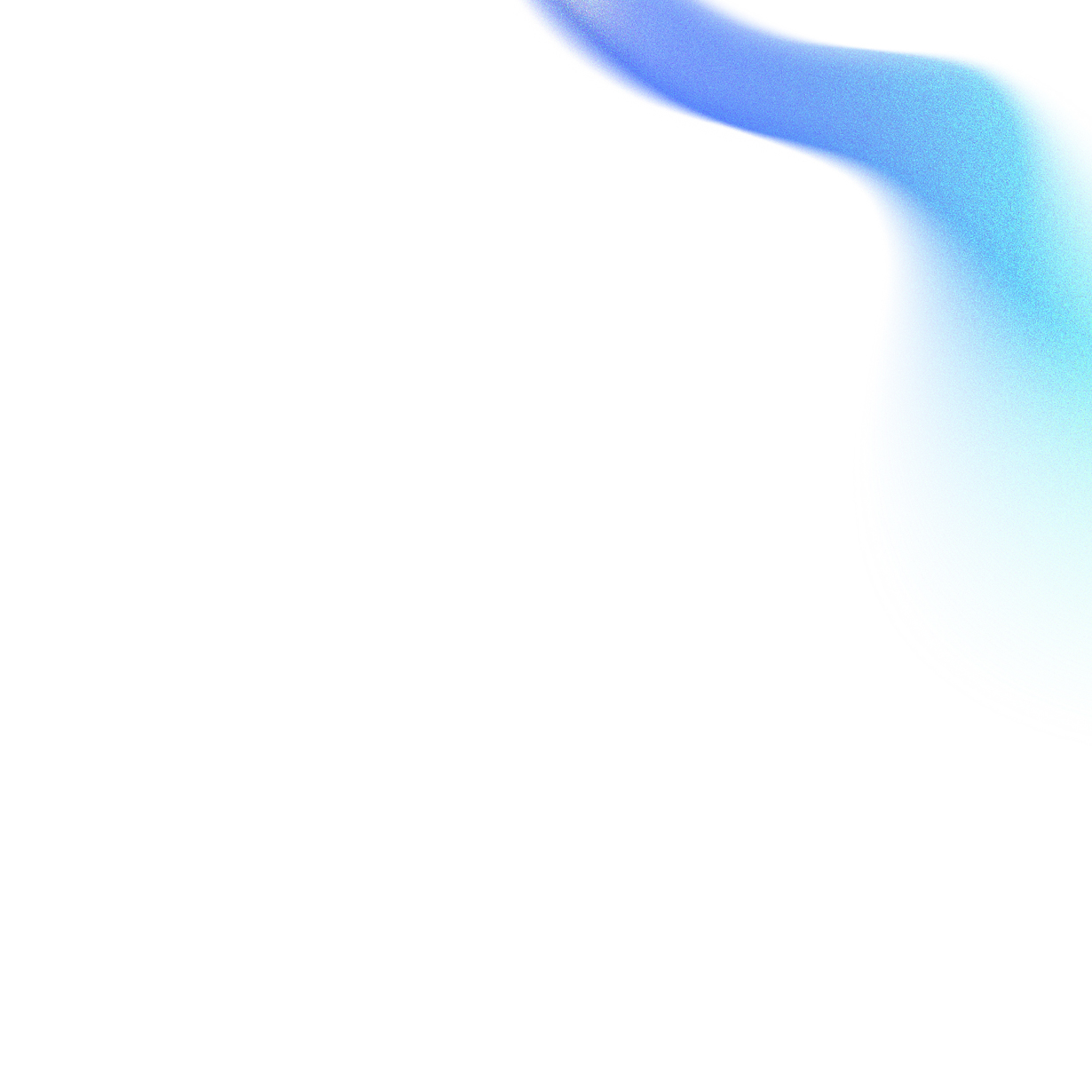
Related Articles
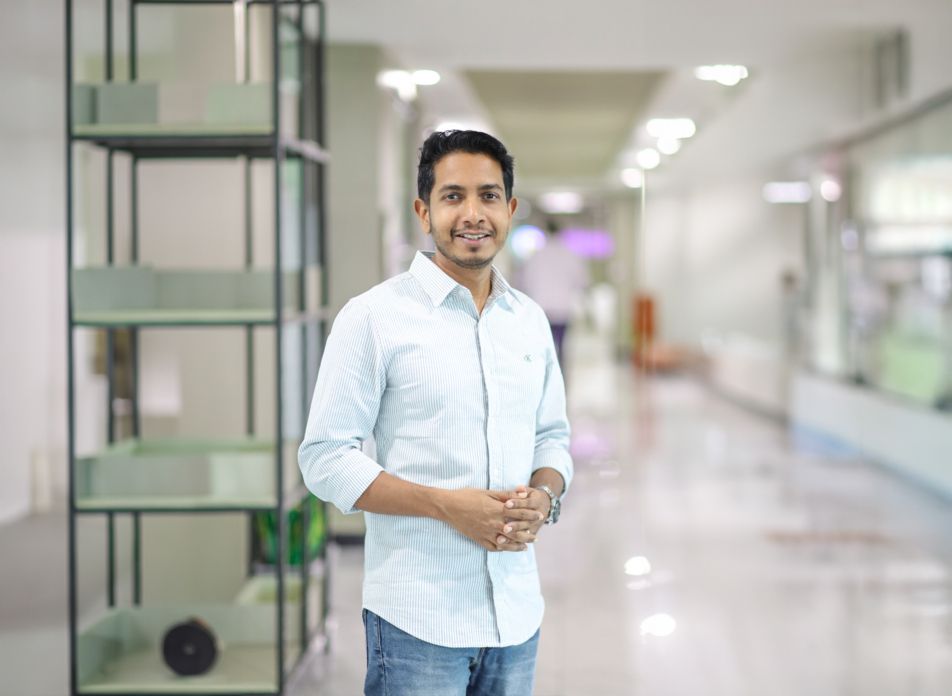