Greetings! I'm Aneesh Sreedharan, CEO of 2Hats Logic Solutions. At 2Hats Logic Solutions, we are dedicated to providing technical expertise and resolving your concerns in the world of technology. Our blog page serves as a resource where we share insights and experiences, offering valuable perspectives on your queries.
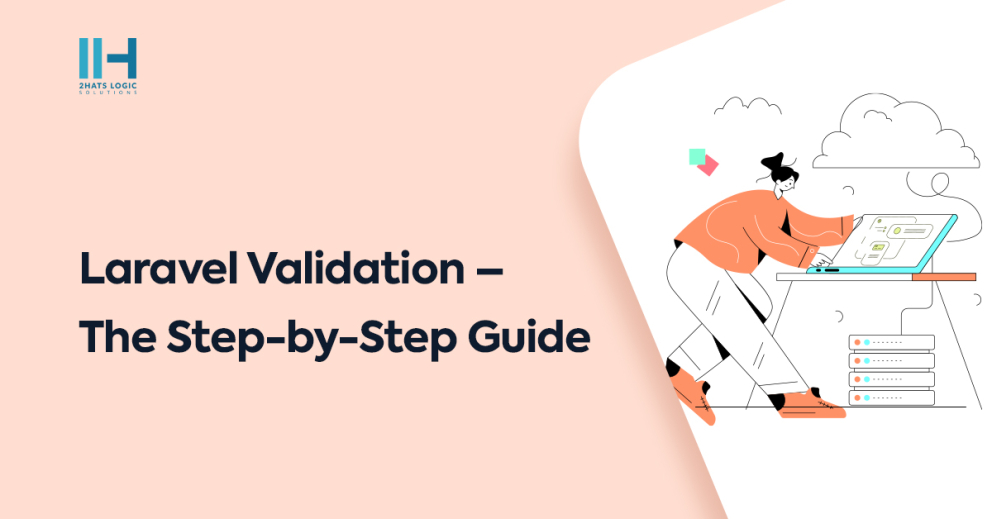
Laravel is a popular and best PHP framework valued for its effectiveness in web development. It places great emphasis on validation—a pivotal aspect in ensuring data integrity and fortifying against vulnerabilities in form handling.
Laravel validation ensures data integrity and security, offering a robust mechanism to validate user inputs and maintain application reliability. Understanding its importance and following the necessary steps guarantees smooth data handling and enhances the overall user experience. This blog explores Laravel validation, highlighting its significance and the essential steps to implement it effectively.
What is Exactly Laravel Validation?
Laravel validation is a process of verifying and ensuring the correctness and security of user inputs in web applications and web stores built with the Laravel PHP framework.
Why is it Important?
It’s crucial for maintaining data integrity and safeguarding against vulnerabilities such as injection attacks and malicious data entry. By validating user inputs, developers can prevent errors, ensure consistency, and protect sensitive information, ultimately enhancing the overall reliability and security of the application.
Step-by-step Guide to Setting Up Validation Rules in Laravel
Let’s look at the steps to set up validation rules in Laravel:
Create a Form
First, create a form in your Laravel application where users can input data. This could be a simple HTML form or a form generated by Laravel’s Blade templating engine.
Define Validation Rules
In your Laravel application, navigate to the relevant controller where you handle form submissions. In the controller method responsible for processing the form data, define validation rules using Laravel’s validation syntax. You can do this by using the validate()
method provided by Laravel.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | ```php use IlluminateHttpRequest; public function store(Request $request) { $validatedData = $request->validate([ 'name' => 'required|string|max:255', 'email' => 'required|email|unique:users|max:255', 'password' => 'required|string|min:8|confirmed', ]); // Process validated data } |
In this example, we’re validating three fields: name
, email
, and password
. The validate()
method will automatically redirect the user back to the form with validation errors if validation fails.
Validation Rules
Let’s break down the validation rules used in the example:
-
required
: The field must be present in the request data. -
string
: The field must be a string. -
max:255
: The field’s length must not exceed 255 characters. -
email
: The field must be a valid email address format. -
unique:users
: The field’s value must be unique in the specified table (users
table in this case). -
min:8
: The field’s length must be at least 8 characters. -
confirmed
: The field’s value must be confirmed by another field with a_confirmation
suffix, e.g.,password_confirmation
. Display Validation Errors
In your form view, you can display validation errors using Laravel’s Blade syntax. For example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | ```html @if ($errors->any()) <div class="alert alert-danger"> <ul> @foreach ($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> </div> @endif |
This code will display a list of validation errors if any are present.
That’s it! You’ve set up validation rules in Laravel using the validate()
method in your controller. This helps ensure that the data submitted through your forms meets your specified criteria before processing.
To display an error under each field you can do like this:
1 2 3 4 5 6 7 | <input type="text" name="firstname"> @if($errors->has('firstname')) <div class="error">{{ $errors->first('firstname') }}</div> @endif |
Custom Validation Rules
Laravel allow developers to define their logic beyond Laravel’s built-in rules. Using closures or custom rule objects, Laravel developers can create rules tailored to their specific needs.
Closures offer a quick and concise way to define simple validation logic inline, while custom rule objects provide a more structured approach for complex rules with reusable logic. With this flexibility, developers can ensure that their applications adhere to unique business requirements and maintain data integrity effectively.
Here is an example of how to create custom validation:
Step-1: Create a Custom Validation Rule Class
Start by creating a custom validation rule class. This class should implement the IlluminateContractsValidationRule interface. This interface requires you to implement the passes and message methods.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 | <?php namespace AppRules; use IlluminateContractsValidationRule; class CustomRule implements Rule { public function passes($attribute, $value) { // Your validation logic goes here // Return true if the validation passes, false otherwise } public function message() { return 'The validation message if the rule fails'; } } |
Step -2: Using Your Custom Validation Rule
You can use your custom validation rule in your controller’s validation logic or form request classes. Simply instantiate your custom rule class and add it to the validation rules array.
1 2 3 4 5 6 7 8 9 | use AppRulesCustomRule; $validatedData = $request->validate([ 'field_name' => ['required', new CustomRule], ]); |
Form Request Validation
Form Request validation in Laravel streamlines the validation process by centralising it within dedicated classes called Form Requests. These classes encapsulate validation logic for specific requests, promoting code organisation and maintainability.
Developers can create custom Form Request classes to define validation rules tailored to each form or API request, keeping the controller methods clean and focused. By using Form Requests, developers can enhance code readability, reduce duplication, and ensure consistent validation across the application, ultimately facilitating robust and efficient validation in Laravel projects.
Validation with AJAX
Validating forms asynchronously using AJAX in Laravel improves user experience by dynamically validating input without requiring a page reload.
Here are the steps to set up AJAX validation:
1. Create a Route
Define a route in your Laravel application to handle the AJAX request.
2. Controller Method
Create a controller method that will be invoked when the AJAX request is made. Within this method, perform the validation logic using Laravel’s validation system.
3. Validation Logic
Inside the controller method, use Laravel’s validation methods to validate the form input data.
4. Return JSON Response
If validation fails, return a JSON response containing the validation errors.
5. Frontend Setup
On the frontend, use JavaScript to capture the form submission event. Instead of submitting the form directly, send the form data to the server via AJAX.
6. AJAX Request
When the user submits the form, use JavaScript to send an AJAX request to the route defined in step 1, passing the form data.
7. Handle Response
Handle the response from the server in the JavaScript code. If there are validation errors, display them to the user dynamically without reloading the page.
Testing Laravel Validation
Testing Laravel validation involves verifying that your validation rules are functioning as expected. This ensures that your application accepts valid data and rejects invalid inputs appropriately. Laravel provides tools like PHPUnit to facilitate testing. You can write test cases to cover various scenarios, including both valid and invalid input data. These tests help maintain the integrity and security of your application, improve user experience, and ensure that your business logic is correctly enforced. By thoroughly testing Laravel validation, you can identify and fix issues early in the development process.
Conclusion
In conclusion, Laravel validation is indispensable for Laravel developers in safeguarding data integrity and fortifying against security vulnerabilities. The outlined process, encompassing custom rule creation, Form Request validation, and AJAX validation, empowers developers to craft resilient applications that prioritize user experience. Rigorous testing with tools like PHPUnit ensures validation rules function seamlessly. If you encounter any difficulties in implementing these practices, consider consulting with a Laravel development services or you can hire a Laravel developer for further consultations. By adhering to these best practices, Laravel developers can confidently deliver high-quality applications that meet stringent standards for reliability and security, thereby enhancing user trust and satisfaction.
FAQ
What is Laravel validation and why is it important?
Laravel validation ensures user input correctness and security in web apps. It's crucial for data integrity and guarding against vulnerabilities like injection attacks, ensuring reliability and security.
How do I set up validation rules in Laravel?
Create a form, define validation rules in the controller, display errors in the view, and optionally create custom rules using closures or rule objects.
Can I create custom validation rules in Laravel?
Yes, by implementing the `IlluminateContractsValidationRule` interface and defining `passes()` and `message()` methods, custom rules can be created for specific business logic or requirements.
What are Form Request classes in Laravel validation?
Form Request classes centralize validation logic for specific requests, promoting code organization and maintainability by encapsulating validation rules within dedicated classes.
How can I validate forms asynchronously (with AJAX) in Laravel?
Define a route to handle AJAX requests, create a controller method for validation, use JavaScript to send form data via AJAX, and handle server responses to dynamically display validation errors without page reloads.
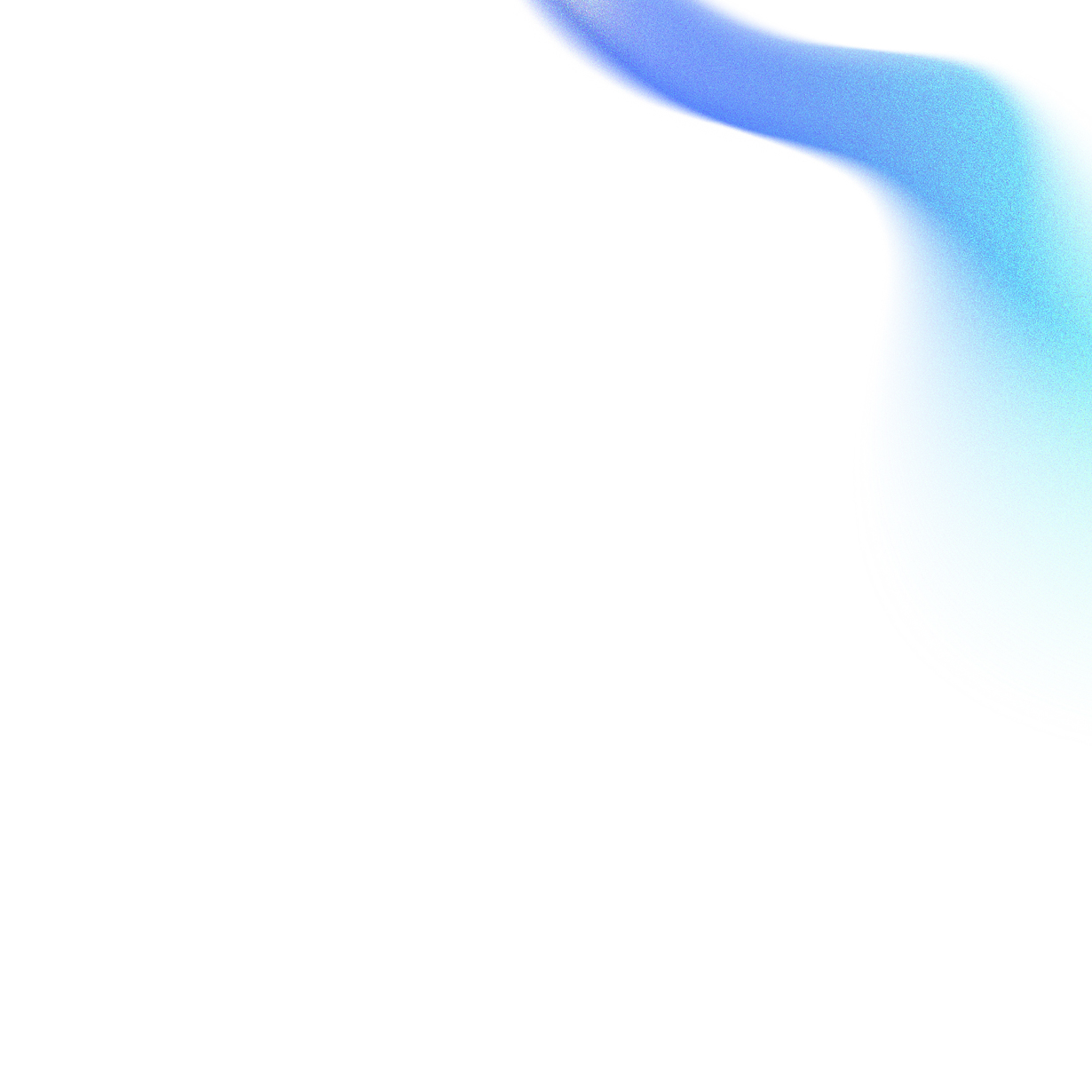
Related Articles
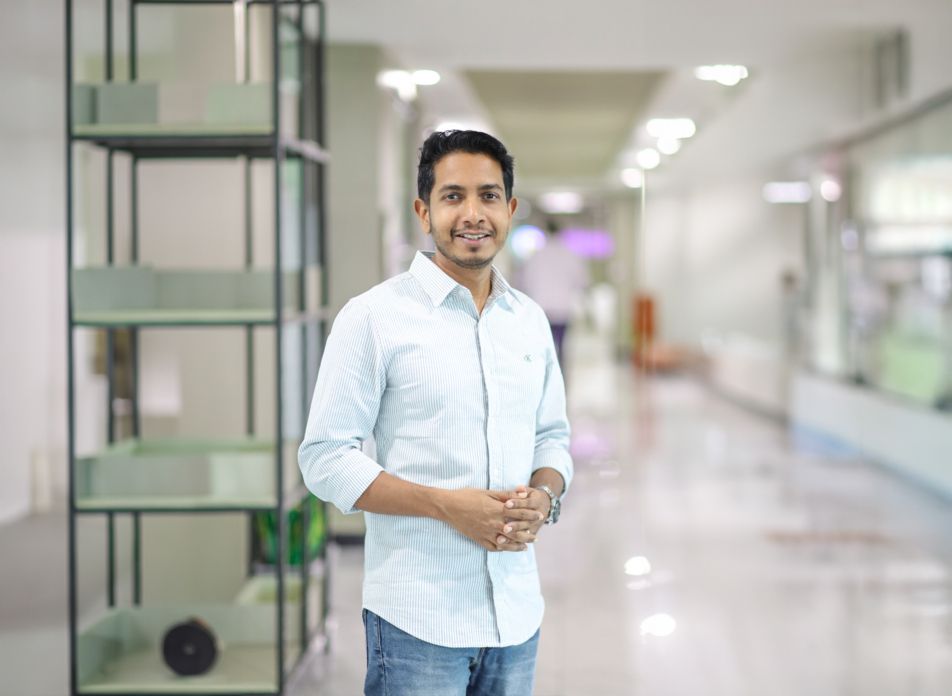