Greetings! I'm Aneesh Sreedharan, CEO of 2Hats Logic Solutions. At 2Hats Logic Solutions, we are dedicated to providing technical expertise and resolving your concerns in the world of technology. Our blog page serves as a resource where we share insights and experiences, offering valuable perspectives on your queries.
Insecure Direct Object References occur when an application provides direct access to objects based on user-supplied input. As a result of this vulnerability, attackers can bypass authorization and access resources in the system directly, for example, database records or files.
Consider, User A uploaded a private photo at http://www.mysite/private/photo/5 and User B uploaded a photo at http://www.mysite/private/photo/6 (you should never use incremental ID’s in the URL in the first place, use some random keys. This is just an example to show the concept.)
Now User B shouldn’t be allowed to view the photo of User A at http://www.mysite/private/photo/5, but many developers miss to address this issue.
This is a common vulnerability that occurs in our projects due to lack of authorization. Most of the time we only check if a user is logged in to view the URL. A user can edit other users data by editing the URL. To prevent this we need to check if a user has access to edit these data.
Solution
The best method to prevent insecure direct object reference vulnerability in laravel is to use a middleware to check if the user has access to the object.
For the above private photo example, we can create a middleware named AccessPrivatePhoto and add this middleware to the route.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | namespace AppHttpMiddleware; use Closure; use AppModelsPhoto; use Auth; class AccessPrivatePhoto { /** * Handle an incoming request to whether the user has permissions to access a Photo * * @param IlluminateHttpRequest $request * @param Closure $next * @return mixed */ public function handle($request, Closure $next) { if(Auth::user()->role() != 'admin') { $photo = Photo::with('user')->find($request->photo); if($photo) { if($photo>user->id != Auth::id()) { return redirect('/client/photos)->with('error', 'You do not have neccessary permissions to access the page'); } } } return $next($request); } } |
This means if a user tries to enter a different URL when logged in it will be checked and if it doesn’t belong to them they are redirected.
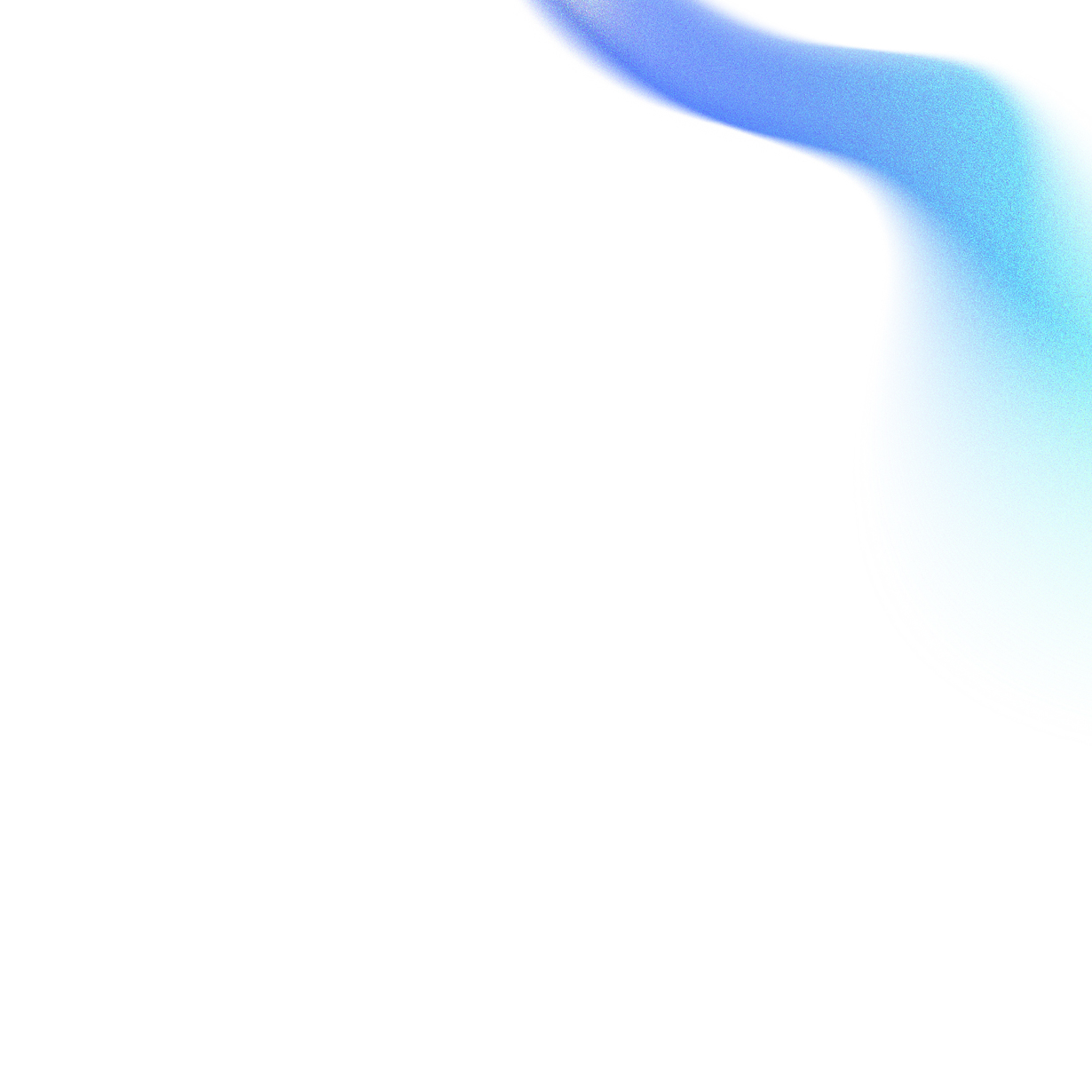
Related Articles
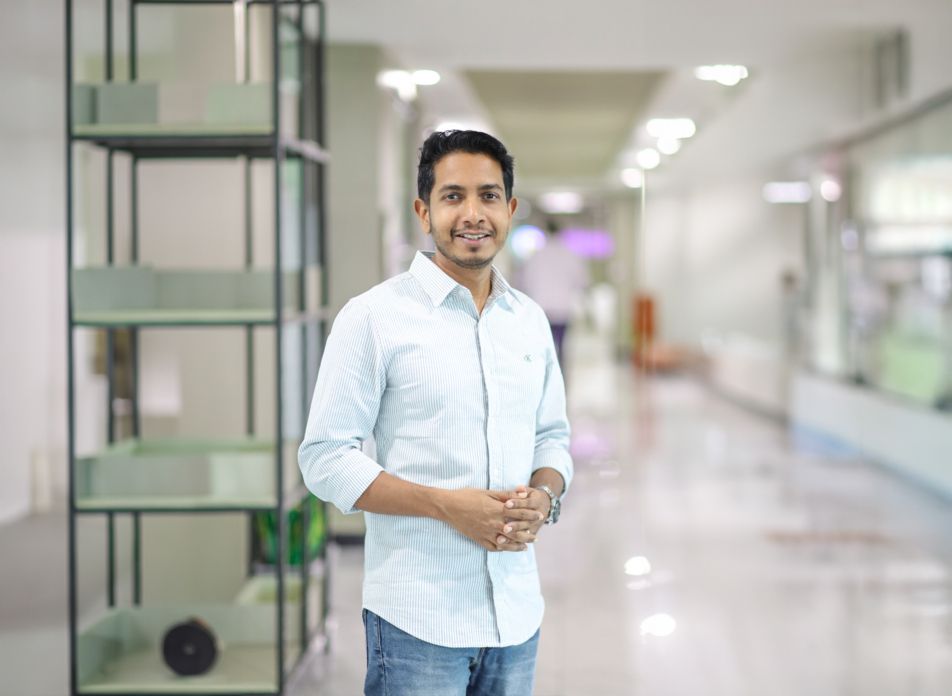