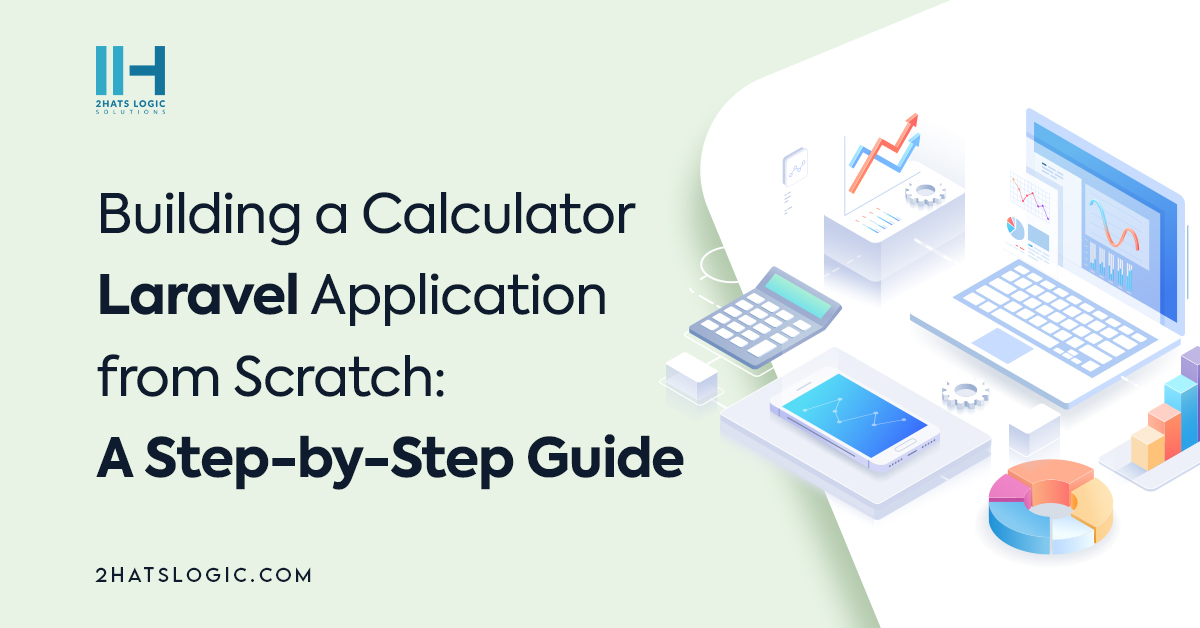
Building a Calculator Laravel Application from Scratch: A Step-by-Step Guide
In the world of web development, learning by doing is a powerful approach than any other method. One way to gain hands-on experience with a PHP framework like Laravel is by building practical applications that gives an idea of core concepts of the framework. In this step-by-step guide, we’ll embark on a journey to create a fully functional calculator application using the Laravel framework. By the end of this tutorial, you’ll not only have a working calculator application but also a solid grasp of Laravel’s fundamentals.
Throughout this guide, we’ll explore the process of setting up a new Laravel project, creating models and controllers, designing user interfaces using Blade templating, and implementing calculator logic. This tutorial will offer valuable insights into the world of Laravel application development.
Let’s get started on our journey to build a calculator Laravel application from scratch!
Step 1: Setting Up Laravel Project.
For setting up laravel project in your system you needs to ensure whether composer and laravel installer already installed. If not install both and if it is complete we can create a new laravel project by running the following command. Here I am using calculator-app for this app you can replace.
‘laravel new calculator-app’
After creating the project please navigate to calculator-app folder by using the following command.
‘cd calculator-app’
In the browser to view this application use the following command and then will provide a local development URL (http://127.0.0.1:8000), In this we can access our laravel project.
‘php artisan serve’
Step 2: Designing the User Interface
let’s focus on creating a user-friendly interface for our calculator application. In this step, we’ll design the views using Laravel’s Blade templating engine.
Create Blade File: Laravel provides a clean and efficient way to organize views. Inside the resources/views directory, create a new folder for our project named calculator.
Design Form: Inside the calculator folder create a new file named calculator.blade.php for a form in which we can input data for calculation.
<form action="{{ route("calculate') }}" method="POST"> @csrf <input type:"text" name="operand1" placeholder="Enter the first number" required> <select name="operator" required> <option "add">+</option> <option "subtract">-</option> <option "multiply">*</option> <option "divide">/</option> </select> <input type:"text" name="operand2" placeholder="Enter the second number" required> <button type="submit">Calculate</button> </form>
This will be the output for the form
Display Result: For displaying the result of calculation we can create another view file with name result.blade.php.
<h1>Calculator Result</h1> <p>Result: {{ $result }}</p> <a href="{{ route('calculator') }}">Back to Calculator</a>
And output for the result page will be
Controller Methods: In controller (which we’ll create in the next step), we can define the ways to handle rendering the views and the calcualtion processes.
Step 3: Building the Controller
Controllers in Laravel act as intermediaries between routes(which we’ll create in the next step) and views, facilitating the flow of data and user interactions. With our user interface designed, now we can create the controller that will handle the logic for our calculator application.
For creating controller in laravel use the following command
‘php artisan make:controller CalculatorController’
This will create a new file named calculatorcontroller.php in the app/Http/Controllers directory. After creating this define mainly two funtions in which one is for showing the calculator and one is for performing the calculator operations and to display the result.
<?php namespace App\Http\Controllers; use App\Http\Controllers\Controller; use Illuminate\Http\Request; use App\Models\Calculator; // Assuming this is the namespace for Calculator model class CalculatorController extends Controller { public function showForm() { return view('calculator.calculator'); } public function calculate(Request $request) { // Validate form input $validatedData = $request->validate([ 'operand1' => 'required|numeric', 'operand2' => 'required|numeric', 'operator' => 'required|in:add,subtract,multiply,divide', // Add other operators here ]); // Create a new Calculator instance $calculator = new Calculator($validatedData); // Perform calculation based on the selected operator $result = $calculator->{$validatedData['operator']}(); // Return the result view with the calculated result return view('calculator.result', ['result' => $result]); } }
we’re creating an instance of the calculator model in this which will create in the step 5.
Step 4: Setting Up Routes
In the routes/web.php file, we can define routes to render the calculator form and display the result. In this, we needs to make sure we have defined the routes to map to the appropriate controller functions.
<?php use Illuminate\Support\Facades\Route; use App\Http\Controllers\CalculatorController; Route::get('/', [CalculatorController::class, 'showForm'])->name('calculator'); Route::post('/calculate', [CalculatorController::class, 'calculate'])->name('calculate');
Step 5: Implementing the Calculator Logic
In the controller, we’re creating an instance of the calculator model and using dynamic method calls to perform calculations based on the selected operator.
‘php artisan make:model Calculator’
We can create a model in laravel using the following command.
And in the model we can define the operations to perform.
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Calculator extends Model { use HasFactory; protected $fillable = ['operand1', 'operand2', 'operator', 'result']; public function add() { return $this->operand1 + $this->operand2; } public function subtract() { return $this->operand1 - $this->operand2; } public function multiply() { return $this->operand1 * $this->operand2; } public function divide() { return $this->operand1 / $this->operand2; } }
Step 6: Displaying Results
With the calculations now being performed, it’s time to display the results to the user. In this step, we’ll cover how to pass data from the controller to the view and render it for the user.
In the method calculate within the CalculatorController.php file returns a variable $result to the viw file named result.blade.php in the calculator folder. Finally it displays the output for the operation entered by the user.
In this Back to calculator button helps the user to return to the calculator operation page.
Step 8: Styling Application
A well-designed user interface enhances the user experience and makes your application visually appealing. We can style calculator application using custom CSS or using a CSS framework like Bootstrap.
Conclusion
Through this step-by-step guide, you’ve learned valuable skills that extend beyond just creating a calculator – you’ve gained insight into key Laravel concepts, user interface design, controller logic etc,. This is a simple and basic guide for creating a laravel project than performing calculator operation without the database connection. There is some more interesting areas like testing and validations which is not discussed in this. Thank you for joining us on this adventure to build a calculator Laravel application
Leave a Reply