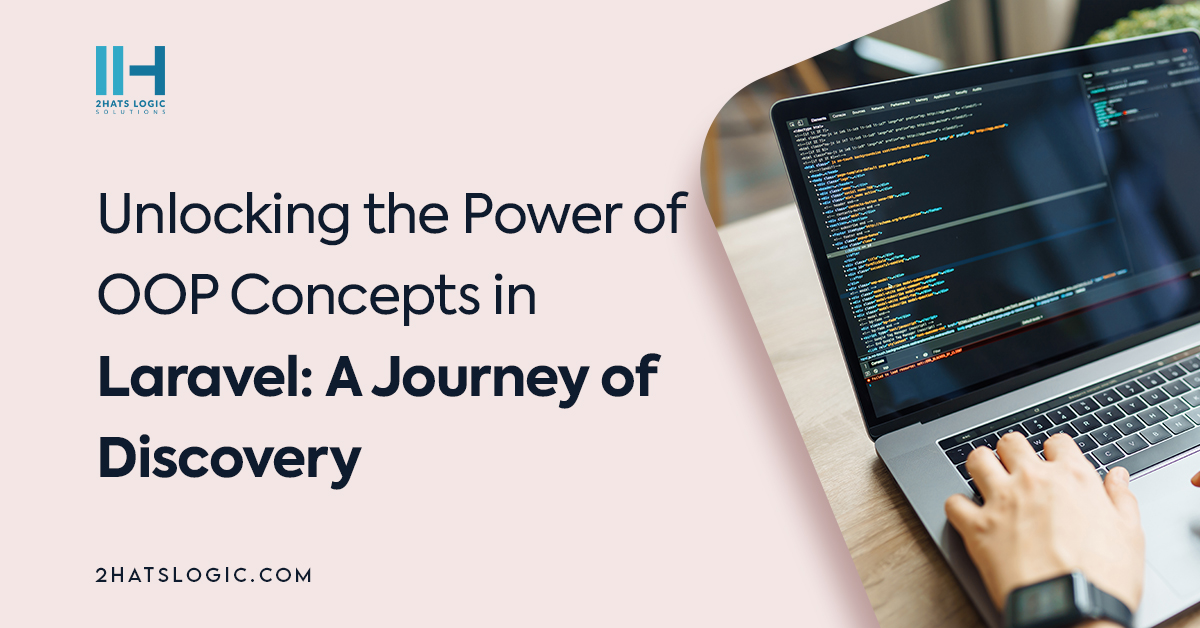
Unlocking the Power of OOP Concepts in Laravel: A Journey of Discovery
Table of contents
Laravel, a PHP web framework, stands out as one of the most popular choices due to its elegant syntax, powerful features, and developer-friendly environment. An essential foundation of Laravel’s success lies in its adept integration of Object-Oriented Programming (OOP) concepts, allowing developers to create scalable, maintainable, organized code.
In this exploration, we’ll delve into how a powerful tool called Laravel can make your web-building journey both fun and productive, all while imaginatively implementing OOP concepts in Laravel. Imagine Laravel as a super-smart helper that speaks the language of web development, making it easy for you to create amazing websites and apps.
What’s the Buzz About OOP?
Before we dive into the Laravel magic, let’s understand some simple ideas that help us build cool stuff. It’s like learning the ABCs before writing great stories!
-
Encapsulation
Imagine you have a treasure chest. You don’t show everyone what’s inside, right? Similarly, we group information and actions together in a ‘class’. This keeps things organized and secret until needed.
-
Inheritance
Think of a family tree, where each generation inherits certain traits and characteristics from their parents and ancestors. In the programming world, classes can also have a similar relationship. One class (the child or subclass) can inherit attributes and behaviors from another class (the parent or superclass).
-
Polymorphism
Imagine you have a collection of musical instruments – a guitar, a piano, and a trumpet. Each instrument makes music, but they do it in different ways. In programming, polymorphism allows you to have other classes with the same method name, but each class implements the method differently.
-
Abstraction
Think of a car with an engine, brakes, wheels, and many intricate parts that work together to make it run. However, when you’re driving, you don’t need to understand all the inner workings. You interact with a simplified interface – the steering wheel, pedals, and gears. This is the essence of abstraction – presenting the essential parts while hiding the complex details. Now, let’s see how Laravel uses these ideas to make web development awesome!
Guardians of Integrity: Embracing Encapsulation in Laravel
Imagine your code as a treasure chest. Inside, valuable gems are hidden from view, accessible only through a special key. This concept is encapsulation in Laravel. It’s about securing the inner workings of your code, allowing only authorized access. Just as your hidden gems are shielded, Laravel’s encapsulation keeps your code safe and organized, revealing its brilliance only where needed
Example: User Authentication
In the web world, we often need to do similar tasks, like checking if someone’s allowed to enter a site. Laravel makes this easy with something called ‘facades’. It’s like having a superpower at your fingertips!
Imagine you’re a bouncer at a party, checking invitations. Laravel’s ‘Auth’ facade helps you do this without any fuss:
use Illuminate\Support\Facades\Auth; if (Auth::attempt(['email' => $email, 'password' => $password])) { // A tale of successful authentication } else { // Alas, the authentication journey ends here }
Laravel also lets different parts of your website share common traits. It’s like how a group of friends might all have similar hobbies. This makes things efficient and saves you time!
Building Blocks with a Twist: Exploring Inheritance in Laravel
You might have different types of things, like clothes and gadgets in an online store. With Laravel’s clever trick, you can create a basic model and then make special ones with extra features, like a dress having its own special design.
Laravel’s use of inheritance enhances code organization and reusability. An excellent example of inheritance in Laravel is the concept of Eloquent Models, which interact with the database.
Example: Eloquent Models
In Laravel, you can create a base Eloquent model class that defines common attributes and methods for database interactions. Then, you can create specialized subclasses for specific entities, inheriting the characteristics of the base model.
use Illuminate\Database\Eloquent\Model; class BaseModel extends Model { protected $table = 'base_models'; // Common attributes and methods } class Product extends BaseModel { // Additional attributes and methods for products } class Order extends BaseModel { // Additional attributes and methods for orders }
Here, the Product and Order classes inherit the database interaction methods and attributes from the BaseModel class. This way, you avoid repeating the same code for each model and keep your codebase organized.
Adapting Identities: Exploring Polymorphism in Laravel
Imagine you’re a magician with a versatile hat. Inside, you’ve got a swan, a rabbit, and a squirrel – all with unique tricks. When you say ‘perform,’ they each do their own magic.
This is like polymorphism in programming. It’s about treating different classes as part of a shared family, even though they’re distinct. Just as your creatures perform unique tricks, polymorphism lets you use the same method name for different classes, each doing its special thing.
In Laravel, this magic is real. Your ‘hat’ is Laravel, the creatures are classes, and their tricks are methods. Laravel leverages polymorphism to create flexible and adaptable code. One notable example of polymorphism in Laravel is the usage of Eloquent relationships.
Example: Eloquent Relationships
In Laravel, you can define relationships between models, such as one-to-many or many-to-many relationships. The beauty lies in the fact that you can treat related models as instances of the same method.
class Comment extends Model { public function commentable() { return $this->morphTo(); } } class Article extends Model { public function comments() { return $this->morphMany(Comment::class, 'commentable'); } } class Video extends Model { public function comments() { return $this->morphMany(Comment::class, 'commentable'); } }
In this example, the Comment model has a polymorphic relationship with both the Video and Article models. This means you can use the same comments method to retrieve comments for both videos and articles, even though they are different types of objects.
Simplifying Development: Abstraction Techniques in Laravel
Laravel understands that some tasks can be complex. So, it creates shortcuts for you! Imagine you’re calling a friend. Instead of dialing their whole number, you just press their name in your phonebook. Laravel does this with ‘service providers. They help you connect to other things without getting tangled in details. Laravel hides the complicated stuff and gives you simple tools. It’s like having a waiter bring you delicious code without worrying about the kitchen magic.
One prime example is the use of service providers.
Example: Service Providers
Imagine you’re integrating a third-party API service into your Laravel application. Instead of scattering API-related configuration and instantiation throughout your code, you can abstract this functionality within a service provider.
use Illuminate\Support\ServiceProvider; use App\Services\ApiService; class ApiServiceProvider extends ServiceProvider { public function register() { $this->app->singleton(ApiService::class, function ($app) { return new ApiService(config('api.key')); }); } }
With this service provider, you can simply bind the ApiService class and access it throughout your application without worrying about the intricate setup process.
As you journey through the Laravel universe, remember that ‘Object-Oriented Programming in Laravel’ isn’t just a fancy phrase. It’s like the secret sauce that makes your web development journey amazing. With Laravel and OOP, you can create web wonders that make people go ‘wow’!
FAQ
Laravel is a PHP web framework known for its elegant syntax, powerful features, and developer-friendly environment. It has gained popularity due to its ability to create scalable, maintainable, and organized code for building websites and web applications.
Laravel implements encapsulation by using concepts like facades and well-structured classes. For example, Laravel's 'Auth' facade allows developers to perform user authentication without exposing the underlying implementation details.
Laravel uses inheritance to enhance code organization and reusability. An example of this is the Eloquent ORM (Object-Relational Mapping) system. Developers can create a base Eloquent model class that contains common database interaction methods and attributes.
Polymorphism in Laravel is exemplified by Eloquent relationships. By defining relationships between models, developers can treat related models as instances of the same method.
Leave a Reply