Greetings! I'm Aneesh Sreedharan, CEO of 2Hats Logic Solutions. At 2Hats Logic Solutions, we are dedicated to providing technical expertise and resolving your concerns in the world of technology. Our blog page serves as a resource where we share insights and experiences, offering valuable perspectives on your queries.
The Shopware app system provides a seamless and effortless way to extend and customize the platform, empowering developers to leverage well-defined extension points. With Shopware, you have the freedom to focus on the interface between your app and the platform, without getting caught up in the complexities of the underlying system. Whether you prefer a specific programming language or framework, you can utilize your existing expertise to build tailor-made solutions.
The decoupling of Shopware and your app ensures excellent compatibility with the multi-tenant cloud system. By utilizing the Admin API and webhooks for communication, your app can seamlessly integrate with Shopware, facilitating efficient data exchange and event handling. This robust integration approach ensures that your app remains scalable, reliable, and compatible with future updates of the platform. The security of the Shopware app system is paramount. The system follows a permission-based model, allowing your app to request specific permissions to access data within the Shopware instance. This ensures that your app only accesses the necessary data and cannot interfere with or modify sensitive information without proper authorization. Furthermore, the app system enforces strict separation between your app and the Shopware core, preventing unauthorized modifications or tampering with the core system.
Shopware also provides secure APIs, such as the Admin API, ensuring secure communication between your app and the platform. These APIs adhere to industry best practices for authentication, authorization, and encryption, guaranteeing the confidentiality and integrity of data exchanged between your app and the Shopware instance.
Overall, the Shopware app system streamlines the development process, allowing you to create powerful and customized solutions seamlessly integrating with the platform. By leveraging the extensive capabilities of Shopware, utilizing your preferred programming language, and incorporating robust security measures, you can build innovative and secure apps that enhance the functionality and user experience of Shopware-powered online stores.
Communication between Shopware and your app
Shopware communicates with your app exclusively through HTTP requests, allowing you the freedom to choose a tech stack that can handle such requests. Your app defines HTTP endpoints in the manifest file to receive event notifications from Shopware. When a relevant event occurs in the shop, Shopware automatically posts the corresponding data to your specified endpoints. This real-time communication enables your app to stay updated and respond promptly to important events within the Shopware ecosystem.
While processing these events, your app can make use of the Shopware API to retrieve any additional data it requires. The Shopware API provides access to a wide range of information, including inventory, customer details, and orders. By leveraging the Shopware API, your app can enhance its functionality and offer tailored features to Shopware users.
File structure
Create a folder for your application inside the custom/apps directory of your Shopware development installation. This folder will house all the files and resources related to your app. Within the app folder, include a manifest file that defines the details and specifications of your app.
The structure looks like this:
└── custom
├── apps
│ └── MyExampleApp
│ └── manifest.xml
└── plugins
Manifest file
The manifest file, which serves as the central point of your app, defines the interface between your app and the Shopware instance. It allows you to provide essential information about your app, such as its name, version, and description. If you are utilizing an app server, you can also specify the registration URL within the manifest file. Furthermore, this file enables you to define various aspects of your app, including the admin module, custom fields, cookies, and permissions. Acting as a centralized configuration file, it encapsulates important details and functionalities of your app within the Shopware ecosystem.
For more information, you can refer to the Shopware Manifest Reference.
App Server
The app server for the Shopware app system is a crucial component that allows seamless integration of third-party applications and extensions within the Shopware ecosystem. It serves as a bridge between the frontend and backend, handling requests from the client-side and providing the necessary resources and functionality. The app server can be built using various technologies such as Laravel, Node.js, or any other preferred framework. Shopware itself provides a Symfony bundle, which is a collection of pre-configured Symfony components that simplify the development process and ensure compatibility with the Shopware platform. By leveraging the app server, developers can extend the capabilities of Shopware, create custom features, and enhance the overall shopping experience for users.
Registration request
To initiate the registration process for your app, a GET request needs to be made to a specific URL specified in your app’s manifest file. The manifest file, written in XML format, provides essential information about your app and its configuration. Within the manifest, you will find a section dedicated to setup, where you define the registration URL. Here’s an example snippet from the manifest file:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | <?xml version="1.0" encoding="UTF-8"?> <manifest xmlns_xsi="http://www.w3.org/2001/XMLSchema-instance" xsi_noNamespaceSchemaLocation="https://raw.githubusercontent.com/shopware/platform/trunk/src/Core/Framework/App/Manifest/Schema/manifest-2.0.xsd"> <meta> ... </meta> <setup> <registrationUrl>https://my.example.com/registration</registrationUrl> </setup> </manifest> |
When the registration request is made, it will be a GET request containing query parameters. These parameters can be handled and verified automatically by the Symfony Bundle provided by Shopware. This bundle simplifies the verification process, ensuring that the registration is secure and streamlined.
Refer Official Symfony bundle for App Server for more
Storefront
One of the advantages of the Shopware app system is that it handles the Storefront building process, eliminating the need for an external server setup. Instead, you can effortlessly incorporate your modifications into the Storefront by placing your customized files, such as .html.twig, .js, or .scss, within your app’s Resources folder
App Scripts
The Shopware app system offers powerful features like App Scripts and Script Hooks. App Scripts enable the inclusion of custom logic executed within the Shopware stack, allowing for richer and deeply integrated extensions. Script Hooks provide the flexibility to inject code at specific execution points, extending Shopware’s functionality. Together, these features empower developers to create dynamic and tailored experiences that seamlessly integrate with Shopware, enhancing the user experience and meeting unique business needs.
Script hooks
In the Shopware app system, scripts are triggered by “Hooks,” which serve as the entry point for each script. By registering scripts within your app, you can specify which hooks should trigger their execution. When a hook is triggered, your script gains access to the relevant data from the current execution context, allowing you to react to it or perform data manipulation as needed.
To use scripts, refer to Script Hooks Reference.
Scripts
App scripts in the Shopware app system are essentially Twig files executed in a sandboxed environment. These scripts are registered to specific hooks and gain access to the associated hook’s data and pre-defined services. Leveraging this, you can execute custom logic within the script, utilizing the available data and services. This enables seamless integration and customization, allowing you to create dynamic app behaviors efficiently.
└── DemoApp
├── Resources
│ └── scripts // all scripts are stored in this folder
│ ├── product-page-loaded // each script in this folder will be executed when the product-page-loaded
hook is triggered
│ │ └── my-first-script.twig
│ ├── cart
│ │ ├── first-cart-script.twig
│ │ └── second-cart-script.twig // you can execute multiple scripts per hook
│ └── …
└── manifest.xml
Administration
To extend the Shopware Administration, simply overriding and extending Administration components by providing custom JavaScript files in the Resources/administration namespace won’t have any effect. Instead, specific extension points are available for extending the Administration using alternative methods. One such method is adding custom modules to the Administration within your app. These modules are loaded as iframes embedded in the Shopware Administration, where your website is displayed. This approach allows you to seamlessly integrate your custom functionality into the Administration, providing a tailored user experience.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | <?xml version="1.0" encoding="UTF-8"?> <manifest xmlns_xsi="http://www.w3.org/2001/XMLSchema-instance" xsi_noNamespaceSchemaLocation="https://raw.githubusercontent.com/shopware/platform/trunk/src/Core/Framework/App/Manifest/Schema/manifest-2.0.xsd"> <meta> ... </meta> <admin> <module name="exampleModule" source="https://example.com/promotion/view/promotion-module" parent="sw-marketing" position="50" > <label>Example module</label> <label lang="de-DE">Beispiel Modul</label> </module> </admin> </manifest> |
Alternatively, you can use Admin Extension SDK to build Admin Modules. Refer to Admin Extension SDK Documentation
Webhooks
Webhooks in the Shopware app system allow you to subscribe to events happening in Shopware. When an event occurs, a POST request is sent to the specified URL for that event. To utilize webhooks in your app, include a <webhooks> element in your manifest file, as shown below:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | <?xml version="1.0" encoding="UTF-8"?> <manifest xmlns_xsi="http://www.w3.org/2001/XMLSchema-instance" xsi_noNamespaceSchemaLocation="https://raw.githubusercontent.com/shopware/platform/trunk/src/Core/Framework/App/Manifest/Schema/manifest-2.0.xsd"> <meta> ... </meta> <webhooks> <webhook name="product-changed" url="https://example.com/event/product-changed" event="product.written"/> </webhooks> </manifest> |
Shopware app system provides developers with the flexibility to extend and customize the platform according to their specific requirements. By decoupling the deployment of Shopware and the app, developers can leverage the Admin API and webhooks to establish communication between the two. The app’s structure involves creating a dedicated folder with a manifest file that defines the app’s details and specifications. The manifest file serves as the central point of the app, encapsulating important information such as the app’s name, version, description, and capabilities. With the freedom to choose their preferred programming language or framework, developers can leverage their expertise to build customized solutions that seamlessly integrate with Shopware.
FAQ
What is a Shopware app?
A Shopware app is a modular extension or plugin that can be integrated into the Shopware e-commerce platform. It adds specific features or functionality to a Shopware store.
What is the architecture of a Shopware app?
The architecture of a Shopware app follows a modular and extensible design. It typically consists of several components, including controllers, services, models, templates, and configuration files.
What is the role of controllers in a Shopware app?
Controllers handle incoming requests and define the logic for processing those requests. They interact with other components to fetch data, perform actions, and generate responses.
What are services in a Shopware app?
Services in a Shopware app encapsulate reusable business logic and provide functionality to other components. They handle complex operations, interact with databases or external services, and can be accessed by controllers, models, or other services.
What are models in a Shopware app?
Models represent the data structures and database tables used in a Shopware app. They define the properties and relationships between entities, and provide methods for querying and manipulating data.
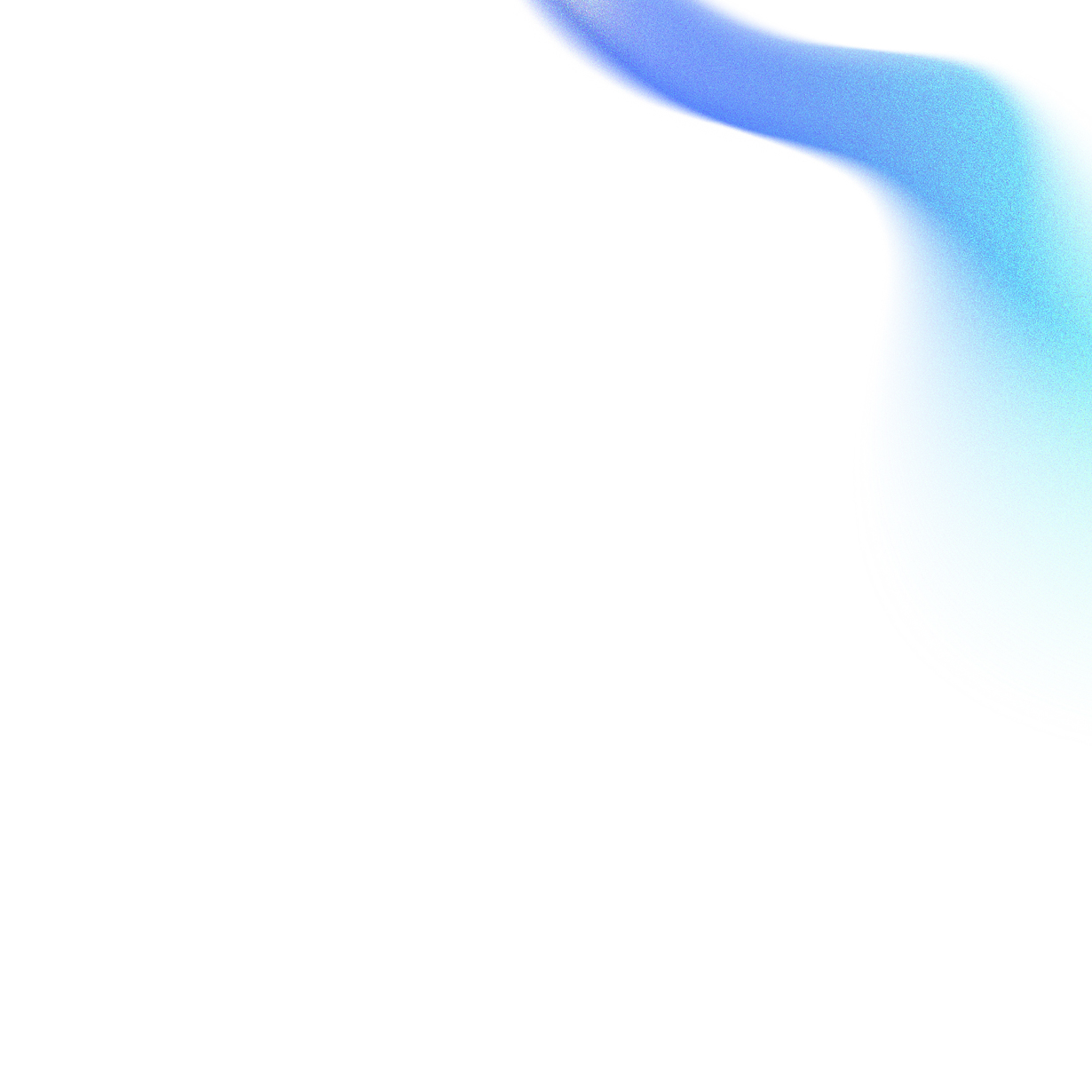
Related Articles
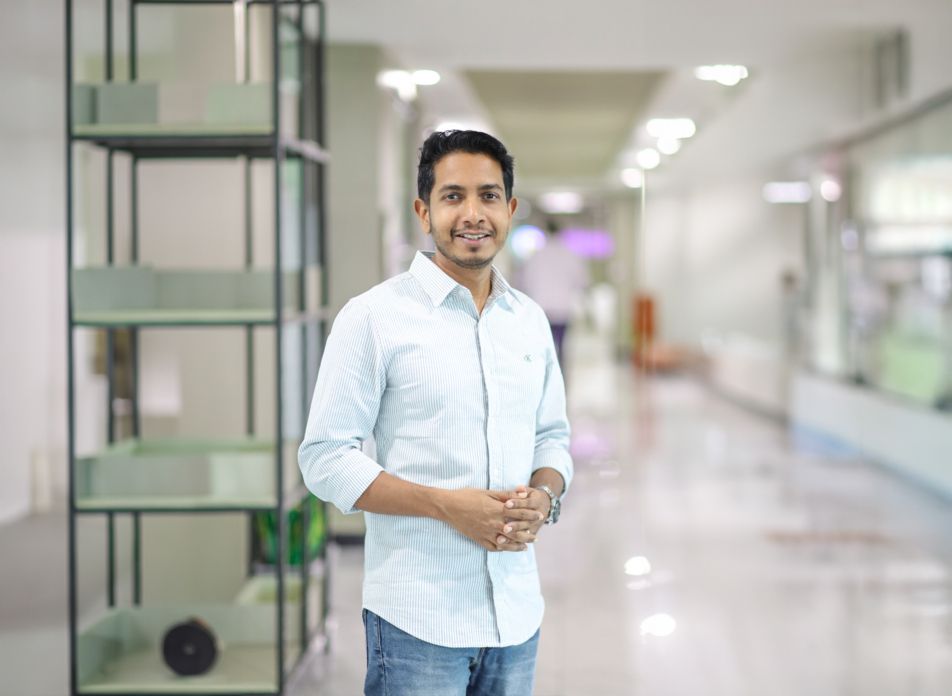