Greetings! I'm Aneesh Sreedharan, CEO of 2Hats Logic Solutions. At 2Hats Logic Solutions, we are dedicated to providing technical expertise and resolving your concerns in the world of technology. Our blog page serves as a resource where we share insights and experiences, offering valuable perspectives on your queries.
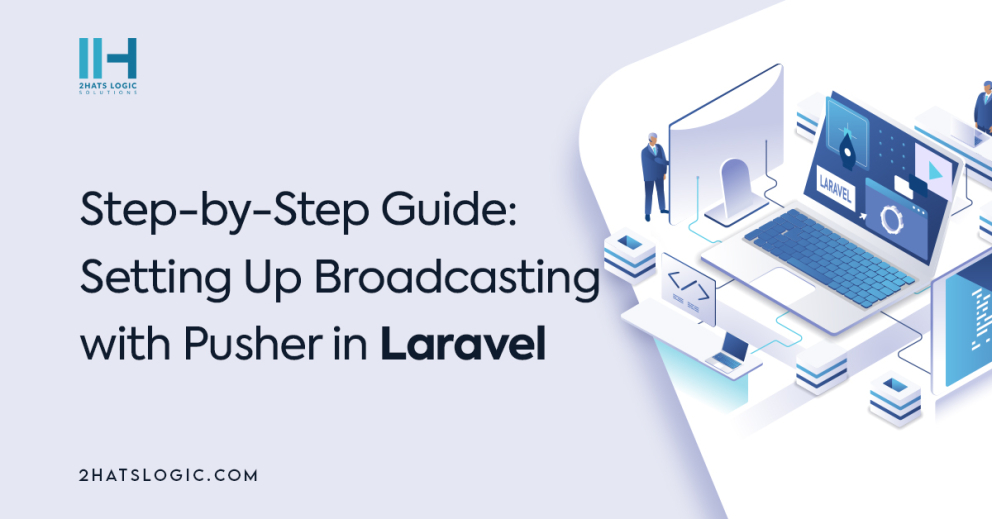
Are you looking to incorporate real-time broadcasting into your Laravel application? Look no further! In this step-by-step guide, we will walk you through the process of setting up broadcasting with Pusher in Laravel. Broadcasting allows you to push server-side events to your users in real time, enabling a more interactive and responsive application. Whether you want to build a live chat feature or display real-time notifications, this guide will help you get started with broadcasting using Pusher in Laravel. So, let’s dive in and enhance the real-time functionality of your Laravel application!
Step 1: Installing Pusher in Laravel
First, navigate to your Laravel project folder in the command line. This step is important as it allows you to access your project files and make the necessary changes. Once you are in the project folder, you can proceed to the next step. Use Composer to install the Pusher package by running the command:
composer require pusher/pusher-php-server.
Composer is a dependency management tool for PHP, and it will automatically download and install the Pusher package for you. This package contains the necessary files and functions that enable you to work with Pusher in Laravel. After the installation is complete, open your project’s .env file. The .env file contains important configuration settings for your Laravel application. In this case, we need to add our Pusher credentials to the .env file. This is done by filling in the following value
PUSHER_APP_ID=your-pusher-app-id
PUSHER_APP_KEY=your-pusher-key
PUSHER_APP_SECRET=your-pusher-secret
PUSHER_APP_CLUSTER=your-pusher-cluster
These credentials are unique to your Pusher account and are required for authentication and message broadcasting. You will get these credentials after taking an account at https://pusher.com/. Remember to save the changes you made to the .env file before proceeding to the next step.
Step 2: Configuring Broadcasting in Laravel
The next step is to configure broadcasting. Broadcasting allows the server to send real-time data to the clients using web sockets. To configure broadcasting in Laravel, open the .env file and set the
BROADCAST_DRIVER=pusher
This tells Laravel to use Pusher as the broadcasting driver for your application.
Finally, open the config/app.php file and uncomment
AppProvidersBroadcastServiceProvider::class,
You will also need to configure and run a queue worker. All event broadcasting is done via queued jobs so that the response time of your application is not seriously affected by events being broadcast. You will also need to configure and run a queue worker. All event broadcasting is done via queued jobs so that the response time of your application is not seriously affected by events being broadcast. By completing these steps, you will have successfully configured broadcasting with Pusher in Laravel, allowing real-time communication between the server and clients.
Step 3: Setting Up Events in Laravel
To set up events run the following command
php artisan make:event MessageEvent
This will create a MessageEvent Class make sure it implements IlluminateContractsBroadcastingShouldBroadcastinterface
My Event class looks like the following:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 | <?php namespace AppEvents; use AppModelsUser; use IlluminateBroadcastingChannel; use IlluminateBroadcastingInteractsWithSockets; use IlluminateBroadcastingPresenceChannel; use IlluminateBroadcastingPrivateChannel; use IlluminateContractsBroadcastingShouldBroadcast; use IlluminateFoundationEventsDispatchable; use IlluminateQueueSerializesModels; class MessageEvent implements ShouldBroadcast { use Dispatchable, InteractsWithSockets, SerializesModels; /** * Create a new event instance. */ public function __construct(public User $user, public string $message = '') { } /** * Get the channels the event should broadcast on. * * @return array<int, IlluminateBroadcastingChannel> */ public function broadcastOn(): array { return [ new PrivateChannel('message-channel.' . $this->user->id), ]; } } |
Declare the properties as public which you want to use in the front-end side.
The new PrivateChannel(‘message-channel.’ . $this→user→id), this is the channel name which you would use in the channels.php file for broadcasting
Step 4: Creating Channels and Broadcasting Messages with Pusher
This is a simple process open channels.php file in the routes directory and add the following code
Broadcast::channel(‘message-channel.{id}’, function (User $user, $id) {
return $user->id == $id;
});
This channel will be only available for the user id which is equal to the value in the $id variable. And the value of this $id will be value from the user id mentioned in the MessageEvent class
Step 5: Configuring and installing pusher in client side
To configure Pusher in the client-side, you first need to include the Pusher JavaScript library in your project. This library allows you to interact with the Pusher service and enables real-time communication. Next, you will need to create a new instance of the Pusher object by passing your Pusher app key as the argument. This app key can be obtained from your Pusher account dashboard. By following these steps, you will be able to configure Pusher in the client-side and utilize its broadcasting capabilities in your Laravel application.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 | npm install --save-dev laravel-echo pusher-js uncomment the following lines in resources/js/bootstrap.js import Echo from 'laravel-echo'; import Pusher from 'pusher-js'; window.Pusher = Pusher; window.Echo = new Echo({ broadcaster: 'pusher', key: import.meta.env.VITE_PUSHER_APP_KEY, cluster: import.meta.env.VITE_PUSHER_APP_CLUSTER ?? 'mt1', wsHost: import.meta.env.VITE_PUSHER_HOST ? import.meta.env.VITE_PUSHER_HOST : `ws-${import.meta.env.VITE_PUSHER_APP_CLUSTER}.pusher.com`, wsPort: import.meta.env.VITE_PUSHER_PORT ?? 80, wssPort: import.meta.env.VITE_PUSHER_PORT ?? 443, forceTLS: (import.meta.env.VITE_PUSHER_SCHEME ?? 'https') === 'https', enabledTransports: ['ws', 'wss'], }); create vue or blade file according to your project, I 'm using laravel vue with interia so my script is following. const messages = ref([]); onMounted(() => { Echo.private(`message-channel.${user.id}`) .listen('MessageEvent', (e) => { console.log(e.message); // e.message wil contains your message messages.value.push(e.message); }); }); |
Step 6: Testing Broadcasting Functionality in Laravel
After setting up broadcasting functionality in Laravel, one way to test it is by creating a test event and broadcasting it.
Creates two routes one for listing the message and another for triggering the broadcast event
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 | Route::get('/message', function () { return Inertia::render('Message');//for listing the broadcast message }); Route::get('/message/{user}', function (User $user) { event(new MessageEvent($user, 'Hello world')); // this will trigger the broadcast event. }); The complete Message.vue file will looks like similar <img src="data:image/gif;base64,R0lGODlhAQABAIAAAAAAAP///yH5BAEAAAAALAAAAAABAAEAAAIBRAA7" data-wp-preserve="%3Cscript%20setup%3E%0A%0Aimport%20AuthenticatedLayout%20from%20'%40%2FLayouts%2FAuthenticatedLayout.vue'%3B%0A%0Aimport%20%7B%20Head%20%7D%20from%20'%40inertiajs%2Fvue3'%3B%0A%0Aimport%20%7B%20usePage%20%7D%20from%20'%40inertiajs%2Fvue3'%3B%0A%0Aimport%20%7B%20computed%2C%20onMounted%2C%20ref%2C%20watch%20%7D%20from%20'vue'%3B%0A%0Aconst%20user%20%3D%20usePage().props.auth.user%3B%0A%0Aconst%20messages%20%3D%20ref(%5B%5D)%3B%0A%0AonMounted(()%20%3D%3E%20%7B%0A%0A%20%20%20%20Echo.private(%60message-channel.%24%7Buser.id%7D%60)%0A%0A%20%20%20%20%20%20%20%20.listen('MessageEvent'%2C%20(e)%20%3D%3E%20%7B%0A%0A%20%20%20%20%20%20%20%20%20%20%20%20messages.value.push(e.message)%3B%0A%0A%20%20%20%20%20%20%20%20%7D)%3B%0A%0A%7D)%3B%0A%0A%3C%2Fscript%3E" data-mce-resize="false" data-mce-placeholder="1" class="mce-object" width="20" height="20" alt="<script>" title="<script>" /> <template> <Head title="Dashboard" /> <AuthenticatedLayout> <template #header> <h2 class="font-semibold text-xl text-gray-800 leading-tight">Dashboard</h2> </template> <div class="py-12"> <div class="max-w-7xl mx-auto sm:px-6 lg:px-8"> <div class="bg-white overflow-hidden shadow-sm sm:rounded-lg"> <div class="p-6 text-gray-900"> {{ user }} <div v-for="(message, index) in messages" :key="index">{{ message }}</div> </div> </div> </div> </div> </AuthenticatedLayout> </template> |
Conclusion
In conclusion, setting up broadcasting with Pusher in Laravel is a straightforward process that can greatly enhance the functionality and interactivity of your application. By following the step-by-step guide, you can easily install Pusher in Laravel, configure it, and set up broadcasting with events and listeners. This enables real-time communication between the server and the clients connected to your application. Additionally, creating channels in Pusher allows you to broadcast messages to specific clients, enabling efficient and interactive communication. By configuring Pusher on the client side, you can listen for events and handle them in real time, further enhancing the functionality of your Laravel application. Finally, testing the broadcasting functionality is essential to ensure that messages are being broadcasted and received as expected. With Pusher and Laravel, you can create robust and interactive web applications that provide a seamless real-time experience for your users. If you encounter any issues or find it difficult to implement hire Laravel developer from a professional Laravel development services provider to get expert solutions.
FAQ
What is broadcasting in a Laravel application, and why would I want to use it?
Broadcasting in a Laravel application involves pushing server-side events to clients in real time, allowing for interactive and responsive features. This is particularly useful for building features like live chat, notifications, and updates that require immediate user interaction.
How do I set up events for broadcasting in Laravel?
Use the php artisan make:event command to create an event class. Ensure that this class implements the IlluminateContractsBroadcastingShouldBroadcast interface. In the event class, define the data you want to broadcast. For example, you can create a MessageEvent class that broadcasts a message.
How do I configure Pusher in the client-side JavaScript?
You need to include the Pusher JavaScript library in your project. Then, create a new instance of the Pusher object using your app key. This key is obtained from your Pusher account dashboard. This configuration allows you to interact with Pusher and handle real-time communication.
How can I test broadcasting functionality in Laravel?
You can create a test event and broadcast it to verify that the functionality is working as expected. For example, you can create routes in your application that trigger the broadcasting of events. This way, you can see the real-time communication in action
Table of contents
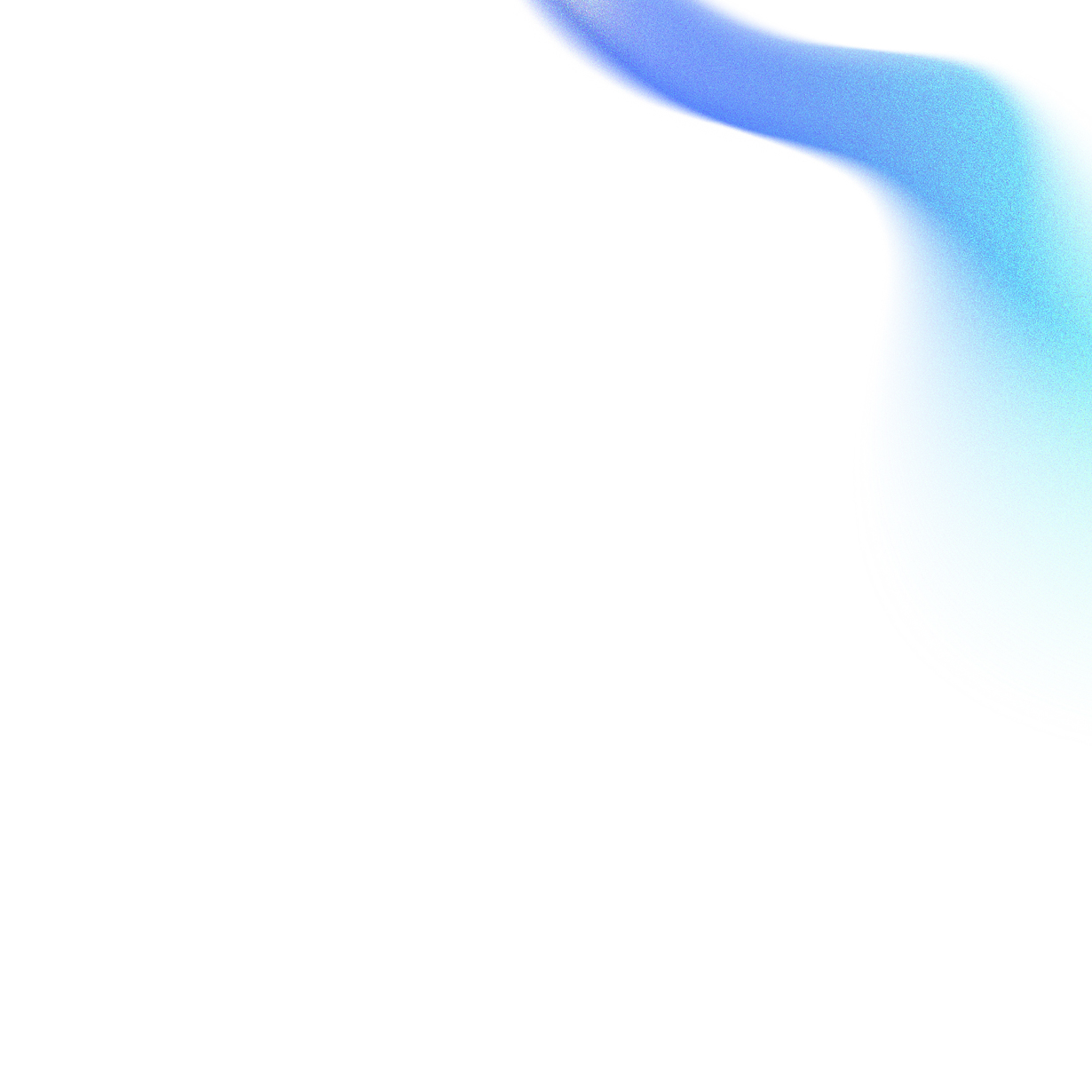
Related Articles
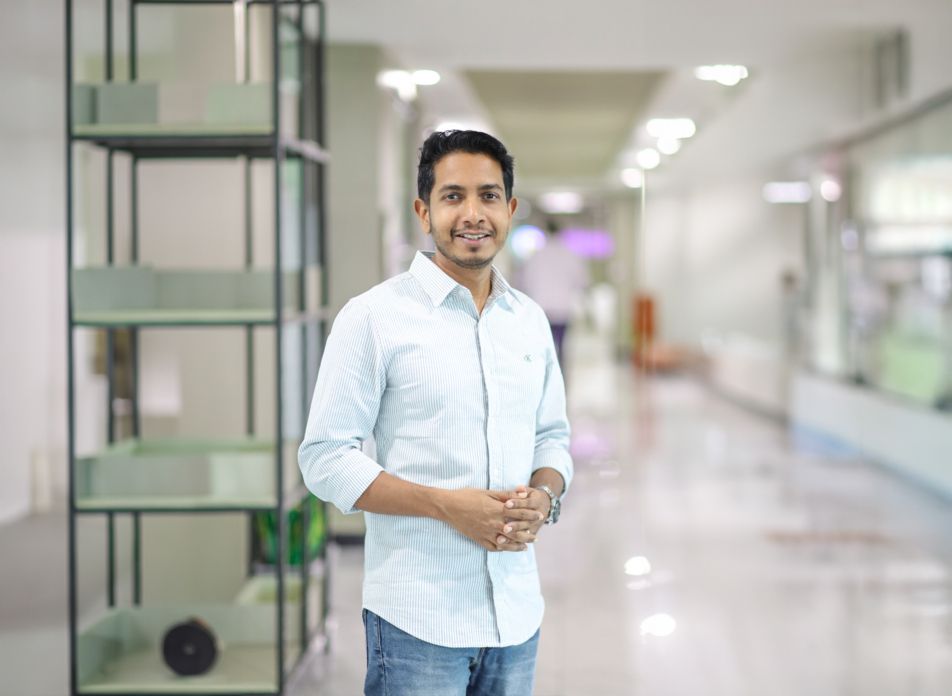