How Do You Handle Missing Request Data Elegantly in Laravel?
When dealing with user input in Laravel applications, not all fields are guaranteed to be present. Hardcoding checks for each possible missing input quickly leads to bloated and repetitive code. That’s where Laravel’s expressive methods like missing() and whenMissing() step in.
These methods allow developers to handle absent data cleanly, whether by applying default values or executing logic conditionally. This approach is especially useful when building flexible settings panels, user preferences, or any form where input may vary.
In this article, we’ll explore how Laravel simplifies handling missing request data and how you can make your controllers cleaner, more readable, and maintainable.
Problem: Managing Optional Inputs in Requests
In many applications, request payloads are dynamic. You might receive full, partial, or no data for a particular field. Manually checking each field with conditional if statements adds unnecessary noise to your codebase.
For example, if a user doesn’t submit their preferences, your app still needs to assign a default theme. Similarly, if email preferences aren’t provided, you may want to fall back on sensible defaults rather than throwing errors or saving null values.
Traditional solutions work, but are verbose. Laravel offers a more elegant way.
Solution: Using missing() and whenMissing()
Laravel’s missing() and whenMissing() methods allow you to define default behavior when certain request fields are absent, without cluttering your code with repetitive checks.
Let’s look at a real-world example of a SettingsController that updates user preferences with defaults when certain inputs are missing.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 | php CopyEdit namespace App\Http\Controllers; use App\Models\User; use Illuminate\Http\Request; class SettingsController extends Controller { public function update(Request $request) { $user = $request->user(); $settings = $user->settings ?? []; // Handle theme with nested defaults $request->whenMissing('theme', function() use (&$settings) { $settings['theme'] = [ 'mode' => 'system', 'color' => 'blue', 'font_size' => 'medium' ]; }, function() use (&$settings, $request) { $settings['theme'] = [ 'mode' => $request->input('theme.mode', 'light'), 'color' => $request->input('theme.color', 'blue'), 'font_size' => $request->input('theme.font_size', 'medium') ]; } ); // Handle notifications with partial updates if ($request->missing('notifications')) { $settings['notifications'] = [ 'email' => true, 'push' => false, 'frequency' => 'daily' ]; } else { $settings['notifications'] = array_merge( $settings['notifications'] ?? [ 'email' => true, 'push' => false, 'frequency' => 'daily' ], $request->notifications ); } // Handle privacy settings with simple default $settings['privacy'] = $request->input('privacy', 'friends_only'); $user->update(['settings' => $settings]); return response()->json([ 'message' => 'Settings updated successfully', 'settings' => $user->fresh()->settings ]); } } |
Input Example (partial data):
1 2 3 4 5 6 7 8 9 10 11 12 13 | json CopyEdit { "notifications": { "push": true } } |
Response:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 | json CopyEdit { "message": "Settings updated successfully", "settings": { "theme": { "mode": "system", "color": "blue", "font_size": "medium" }, "notifications": { "email": true, "push": true, "frequency": "daily" }, "privacy": "friends_only" } } |
Conclusion
Laravel’s missing() and whenMissing() methods are powerful tools for crafting cleaner, more intelligent request-handling logic. They eliminate the need for repetitive conditionals and keep your controller logic focused and readable.
With these expressive helpers, your Laravel application becomes more resilient, maintainable, and user-friendly, even when request data is incomplete.
Explore our Laravel Development Services
Recent help desk articles
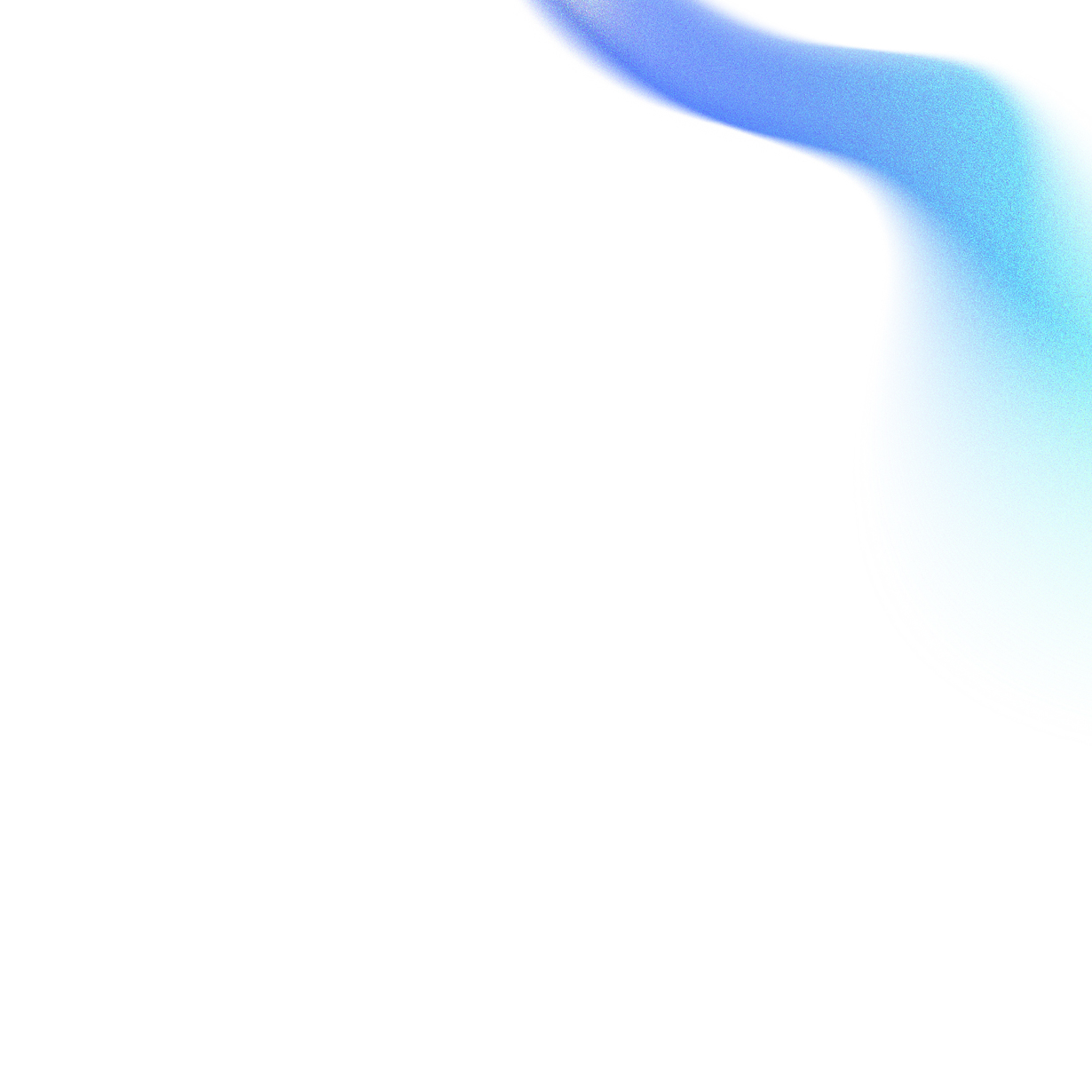
Greetings! I'm Aneesh Sreedharan, CEO of 2Hats Logic Solutions. At 2Hats Logic Solutions, we are dedicated to providing technical expertise and resolving your concerns in the world of technology. Our blog page serves as a resource where we share insights and experiences, offering valuable perspectives on your queries.
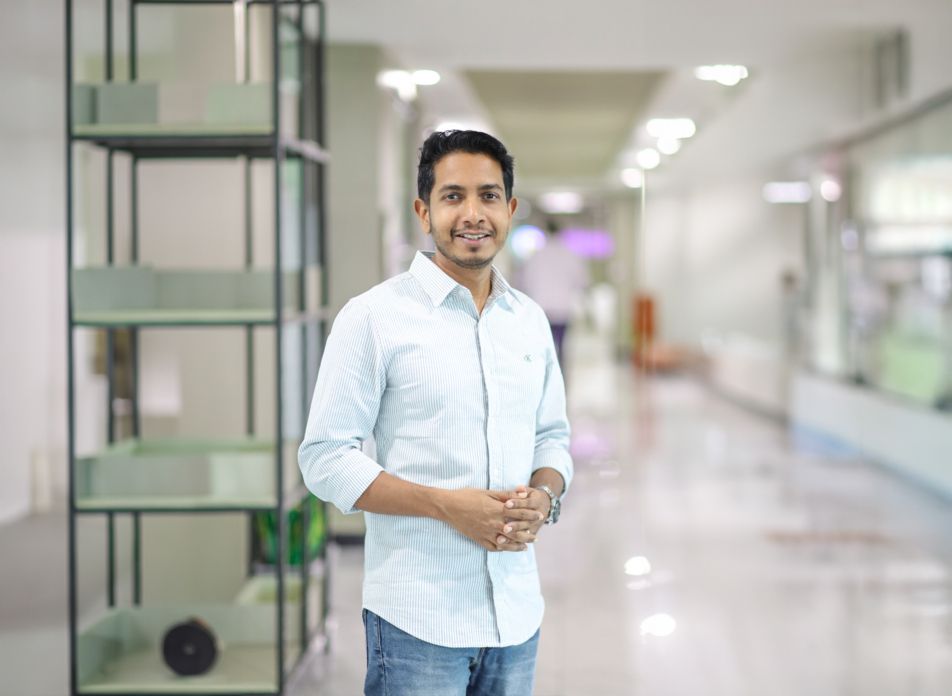