Greetings! I'm Aneesh Sreedharan, CEO of 2Hats Logic Solutions. At 2Hats Logic Solutions, we are dedicated to providing technical expertise and resolving your concerns in the world of technology. Our blog page serves as a resource where we share insights and experiences, offering valuable perspectives on your queries.
Amazon Web Services (AWS) provides a reliable and scalable platform for hosting web applications and storing data. One of the ways to transfer files to and from an AWS server is through the Secure File Transfer Protocol (SFTP). In this guide, we’ll show you how to use Laravel to upload, download, and remove files in an AWS server using SFTP.
To get started, you’ll need to set up an SFTP server on your AWS instance and install the necessary packages in your Laravel application. Once you’ve configured your SFTP connection, you can use Laravel’s built-in Filesystem API to interact with your AWS server.
Below are the steps needed to Upload the file to the AWS server:
- Create a symbolic link in our Laravel project to access our storage/app/public folder publicly. For this run the artisan command
php artisan storage:link
This will create a symbolic link for the storage folder. - After that, we have to install the SFTP driver package using the command
composer require league/flysystem-sftp ~1.0
- Set up SFTP file configuration in our Laravel project. For this add the server details to config/filesystems.php.
SFTP file configuration can be as follows :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | 'sftp' => [ 'driver' => 'sftp', 'host' => 'example.com', // aws server host name 'username' => 'your-username', 'password' => 'your-password', // Settings for SSH key based authentication… // 'privateKey' => '/path/to/privateKey', // 'password' => 'encryption-password', // Optional SFTP Settings… // 'port' => 22, // 'root' => '', // 'timeout' => 30, ], |
- Next, add Laravel Storage extension in the boot() function of app/Providers/AppServiceProvider.php
1 2 3 | Storage::extend('sftp', function ($app, $config) { return new Filesystem(new SftpAdapter($config)); }); |
Before the class definition of AppServiceProvider uses these classes in it :
1 2 3 | use Storage; use LeagueFlysystemFilesystem; use LeagueFlysystemSftpSftpAdapter; |
- The initial setup for file transfer is done. Next is to move the file to the server
Different file transfer methods
- To write the contents of a file to another file in SFTP use the following code
1 | Storage::disk('sftp')->put($destinationFileName, file_get_contents($fileName)); |
$fileName is the file name including path. This file will be in the storage/app/public folder. Files in the publicly accessed folder can be moved to another server.
- Direct file upload using post data can be as follows
1 | Storage::disk('sftp')->putFile($path, $request->file(‘fileName’)); |
1 | Storage::disk('sftp')->putFileAs($path, $request->file(‘fileName’), ‘filename.jpg’); |
- To add content to an existing file
1 | Storage::disk('sftp')->append($path/$fileName, ‘test content’); |
Download the file from the AWS server
To download files from the server use the following code
1 | Storage::disk('sftp')->download($path/$fileName); |
This will download the file and the file name will be the current filename in that server. If we need to change the file name for the downloaded file pass the required file name as the second argument in the download function.
Remove the file from the AWS server
To remove a file from the server use the following code
1 | Storage::disk('sftp')->delete($path/$fileName);<br><br><br><br><br><br><br> |
Conclusion
In this guide, we explored the process of uploading, downloading, and removing files on an AWS server using SFTP in a Laravel application. The key steps involve setting up an SFTP server on your AWS instance, installing the necessary packages in Laravel, and configuring the SFTP connection in the Laravel project.
By following the steps and understanding the provided code snippets, developers can seamlessly integrate SFTP file transfer functionality into their Laravel applications hosted on AWS. This ensures efficient and secure file management, enhancing the overall performance and reliability of web applications. If you encounter any difficulty implementing you can always hire Laravel developer or you can consult with professional Laravel development services to cater to your requirements.
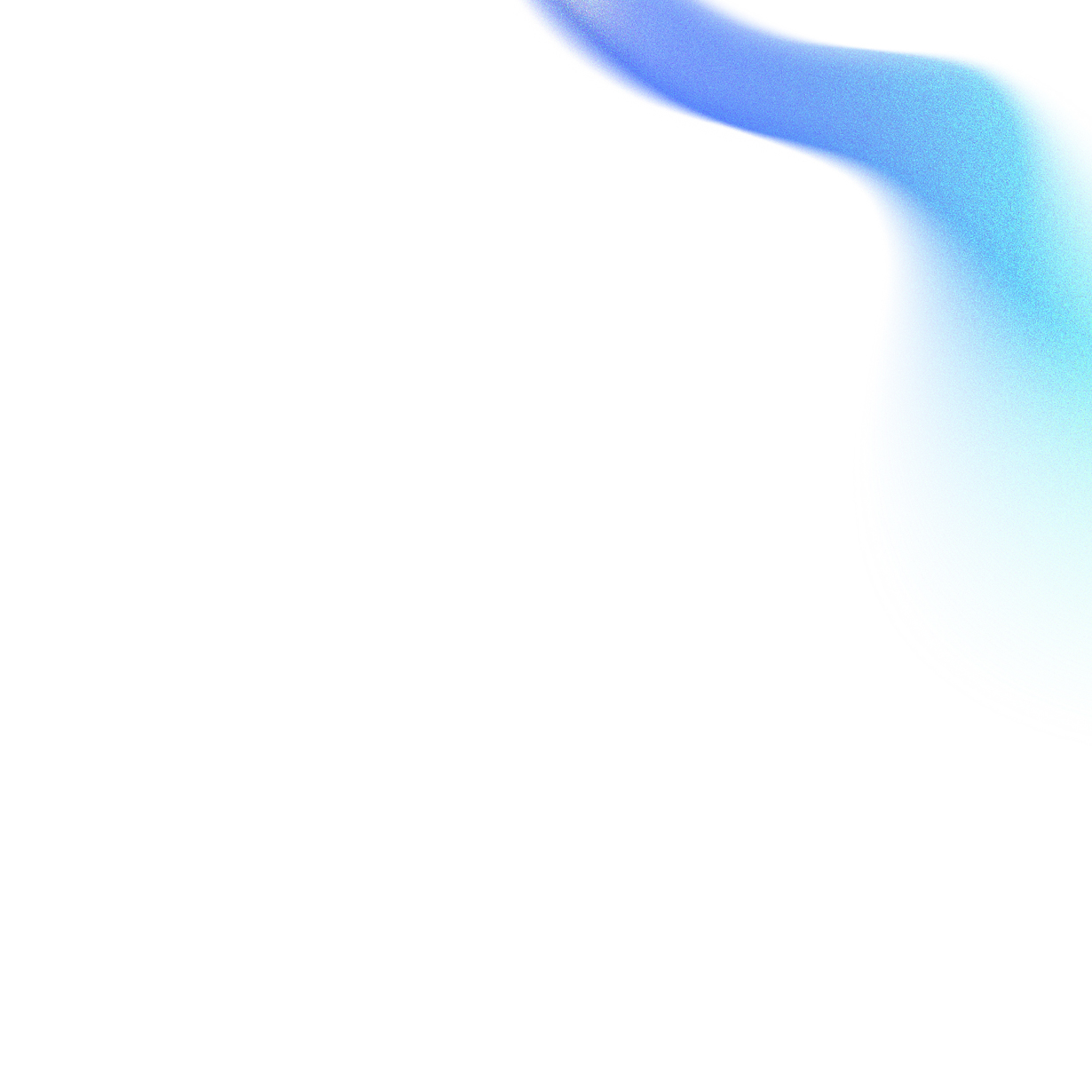
Related Articles
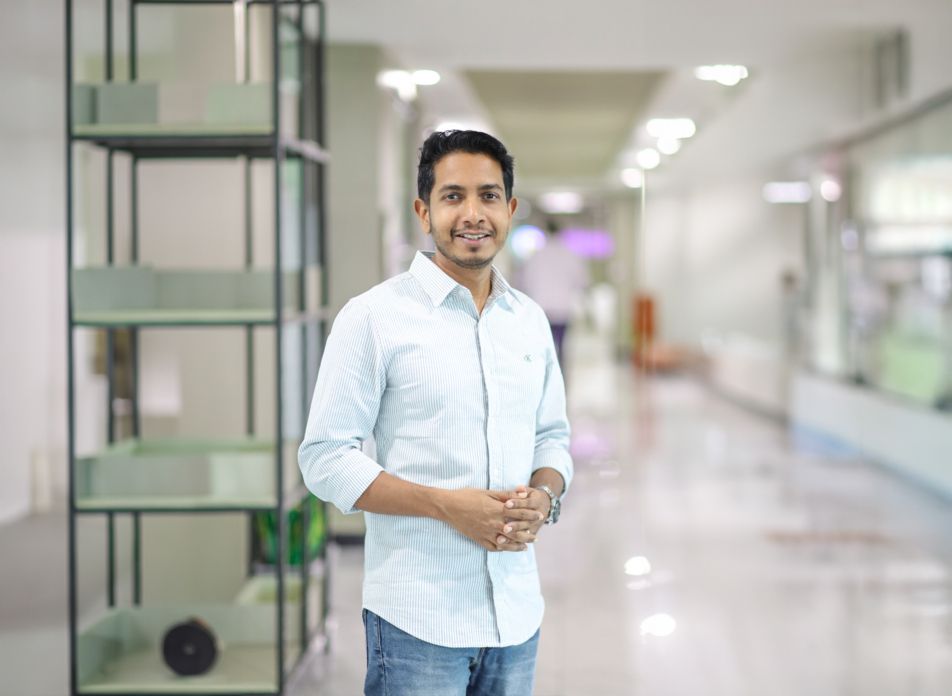